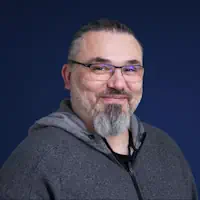
Strapi: How to create a plugin collection and internal API to manage it?
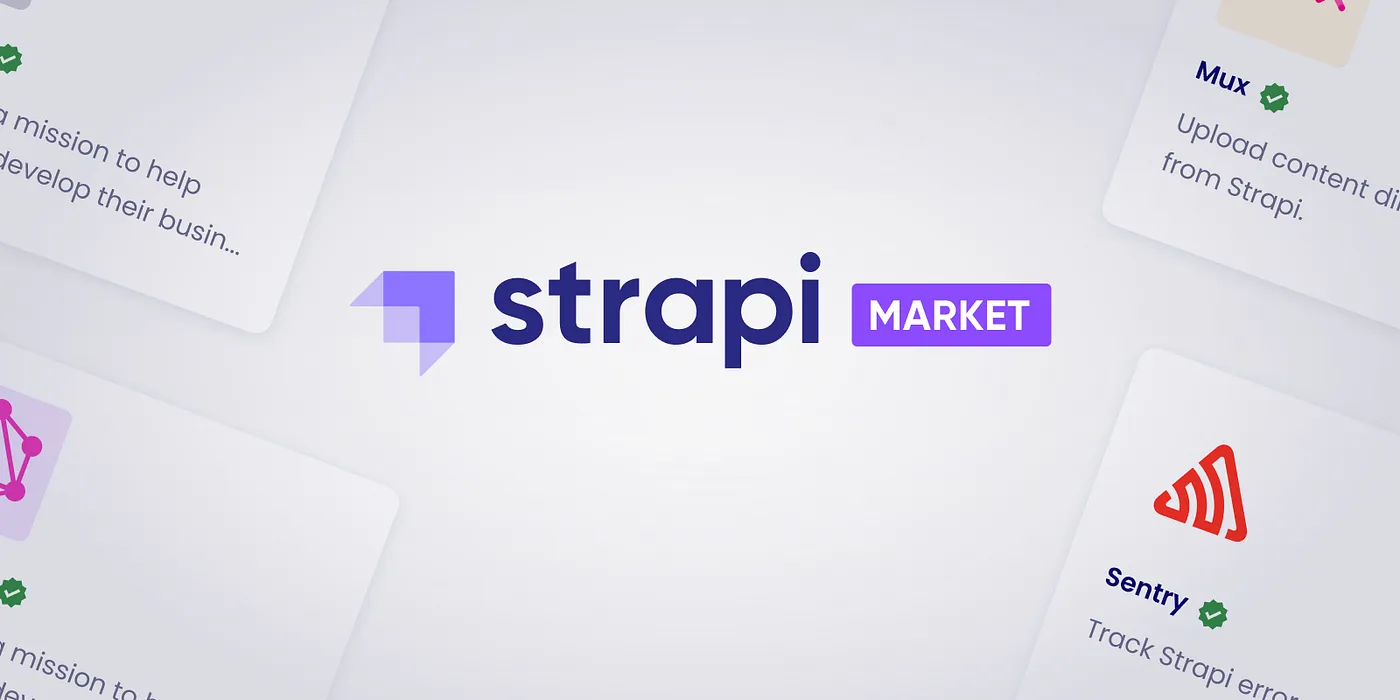
Welcome back!
We'll see how to implement, inside our plugin, a collection and the API to request it.
Create the custom collection
The new collection will be named: help (fantastic name!).
Open a terminal in "api" folder and use the generate command from Strapi.
yarn strapi generate
This time, select Content-Type.
- On "Content-Type display name", input: help
- On "Content-Type singular name and plural", select the default choice with enter key
- On "Choose the module type", select the Collection Type
- On "Use draft and publish", select No
- On "Do you want to add attributes", select Y
- On "Name of attribute", input: "key" and select a string type
- On "Do you want to add another attribute", select No
- On "Where do you want to add this model", select "Add model to existing plugin" and select "awesome-help"
- On "Bootstrap API related files", select Yes.
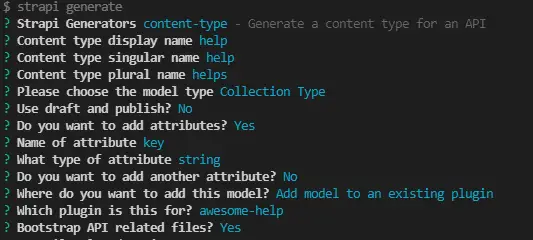
Yarn strapi generate: all steps resumed
Strapi generates all files we need to implement for the "help" custom collection.
For our custom Content-Type, you'll notice that Strapi generates all files inside "api/src/plugins/awesome-help/server" folder.
Let's go inside "api/src/plugins/awesome-help/server/content-types/help", you'll find a "schema.json" file.
/*************************** BEGIN TIPS ! ***********************/
Sometimes, you want to hide your custom collection from Content Manager and Content Builder because it's a collection you use to store some internal plugin logical data. Inside the "schema.json" file, you can add some options with the "pluginOptions" object.
The "content-manager" and "content-type-builder" sections include a visible property that allows you to hide or show the collection.
Perfect for debugging internal plugin collection!
/*************************** END TIPS ! ***********************/
This file contains the structure of the custom collection. The properties name of objects in schema is sufficient to understand their meanings. In addition of the schema file, Strapi generates for you a route, a controller and a service for help collection inside these folders:
- "api/src/plugins/awesome-help/server/routes/help.js"
- "api/src/plugins/awesome-help/server/controllers/help.js"
- "api/src/plugins/awesome-help/server/services/help.js"
But, if you launch the Strapi Admin website, the custom collection is not present inside the Content Manager. You need to make some updates.
In the folder "api/src/plugins/awesome-help/server/content-types/help", create a new file "index.js". Open it and update it:
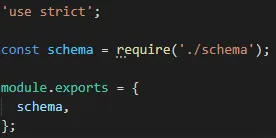
Importation of schema.json
Come back inside "api/src/plugins/awesome-help/server/content-types" and open "index.js" file, update it:
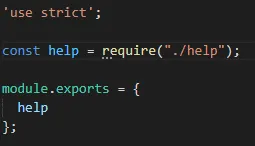
Importation of content-type
Strapi has access to the schema of the custom collection and can use it.
Launch the Strapi Admin Website, in "Content Manager" you will find a help collection!
Click on Help in the middle left menu, the default help list view is now opened. Please look at the address bar and you'll note that the link includes "collectionType/plugin::awesome-help.help".
Yes, you definitively added a custom plugin collection!
Create some entries to test your collection. Strapi automatically generates the text field we defined inside the schema.json structure (attributes section).
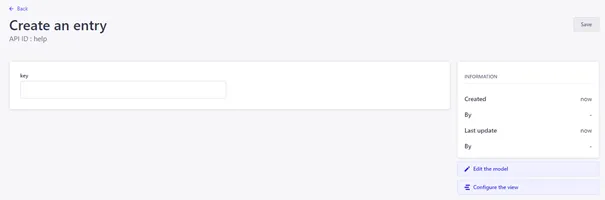
Default form to create a custom help entity
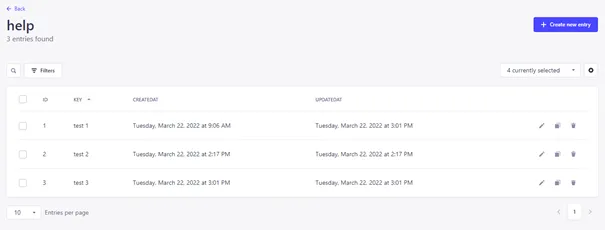
Default list for custom help collection
Create the custom API
Now that the collection is created, we want to interact with it.
As we said previously, Strapi generates a route, a controller and a service.
Route definition
Open the file "api/src/plugins/awesome-help/server/routes/help.js" on update the code with:
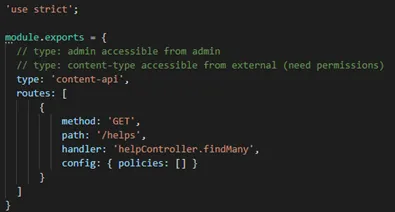
Default route definition
Code explanation: we create a new route with the path "/helps" on a GET request and this route target the "helpController" and use "findMany" function.
Notice the type at the top of the file. This property is VERY important because it determines the behavior of your API. Two values are available for type:
- content-api
- admin
The first one determines that the API can be accessible from external calls like a classical rest API. You need to define some permissions to access it inside the role settings from Strapi Admin Website.
The second one determines that this API is internal and can be accessible only by the admin part (front-end part) of the plugin. You can directly call your API from front-end code, no need to define extra permissions.
In a first time, we use the API in "content-api" type mode to test the API from external calls. Later we'll enable the "admin" type to use API from the admin content.
Come back and edit "api/src/plugins/awesome-help/server/routes/index.js" and edit it:
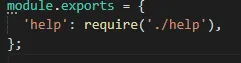
Importation of the custom route
You define a route to the helpController, but this one is not functional, we need to update more files!
Controller definition
Open the file "api/src/plugins/awesome-help/server/controllers/help.js" on update the code with:
Code explanations:
- helpService is a constant who reference the instance of helpService (we define it later :D).
- findMany function is an async function who calls the helpService to request the help entities.
As fot the route, we need to reference the controller. Open the file "api/src/plugins/awesome-help/server/controllers/index.js" and update the code with:
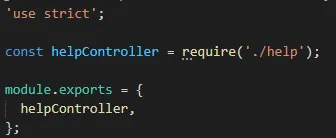
Importation of the custom controller
Now Strapi recognizes the controller!
One more last step and we can use the API. We need to create the service part of the API.
Service definition
Open the file "api/src/plugins/awesome-help/server/services/help.js" on update the code with:
Code explanations:
- query is a constant who reference the instance of Strapi.db.query service. As you see this service is very useful to request the strapi objects repository.
- async findMany function returns the help entities from help collection.
As for the route and the controller, we need to notify Strapi that the service exists:
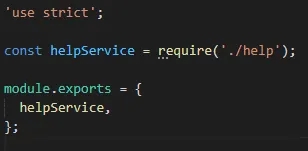
Importation of the custom service
And we're done!
Test the API
The API is now functional, we need to test it.
Open Strapi Admin website and click on settings/roles/public/Awesome-Help and activate the findMany function. Your API is now accessible from an external source.
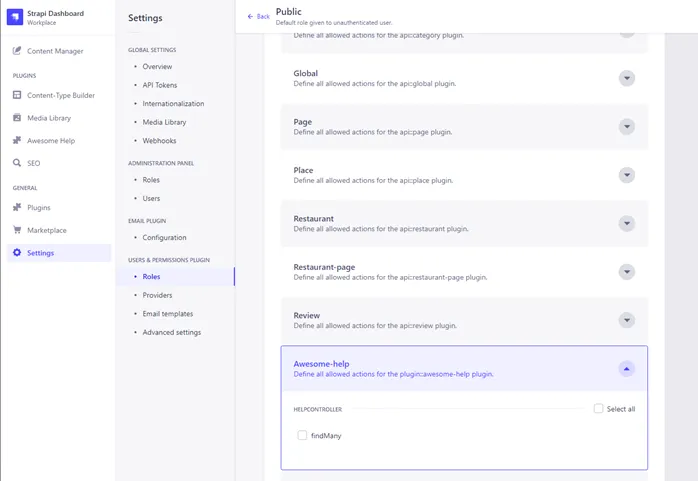
Strapi Admin website: assign a role to public (for all unauthenticated users)
To test the API, we'll use the fantastic POSTMAN.
Create a new request (GET call) on the following path:
http://localhost:1337/api/awesome-help/helps
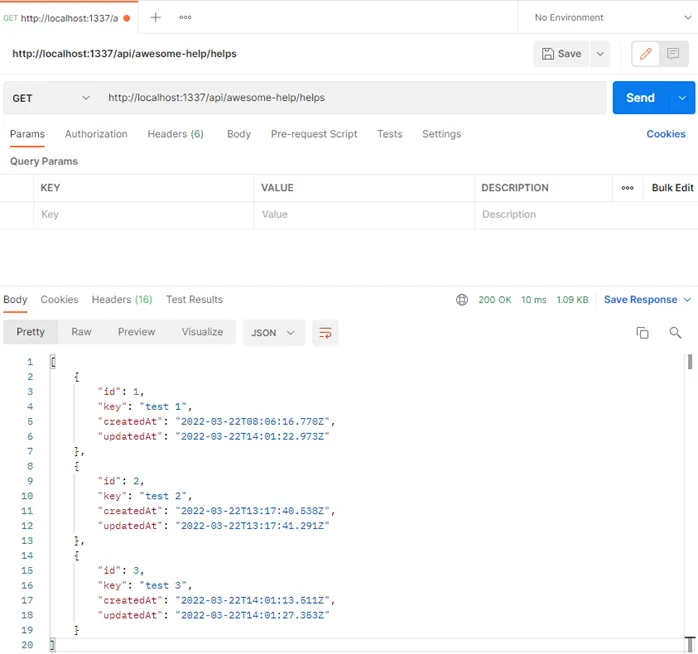
The postam UI: get helps from the custom API
If you add some help entities, the API returns all of them with a beautiful JSON array. Thank you Strapi Team!
Mission complete!
And don't forget our GIT to get the code: https://github.com/ExFabrica/strapi-stories/tree/develop/part-2
See U!